Privileged to be trusted by leading businesses.
We are proud to be a digital solutions partner for global brands and a trusted custom software development company, helping businesses leverage the power of technology.
Our Digital Solutions for Modern Businesses
Website Development
FuturByte delivers a complete suite of web development services. With industry-leading development professionals, we create bespoke web solutions, leveraging modern development practices and software languages.

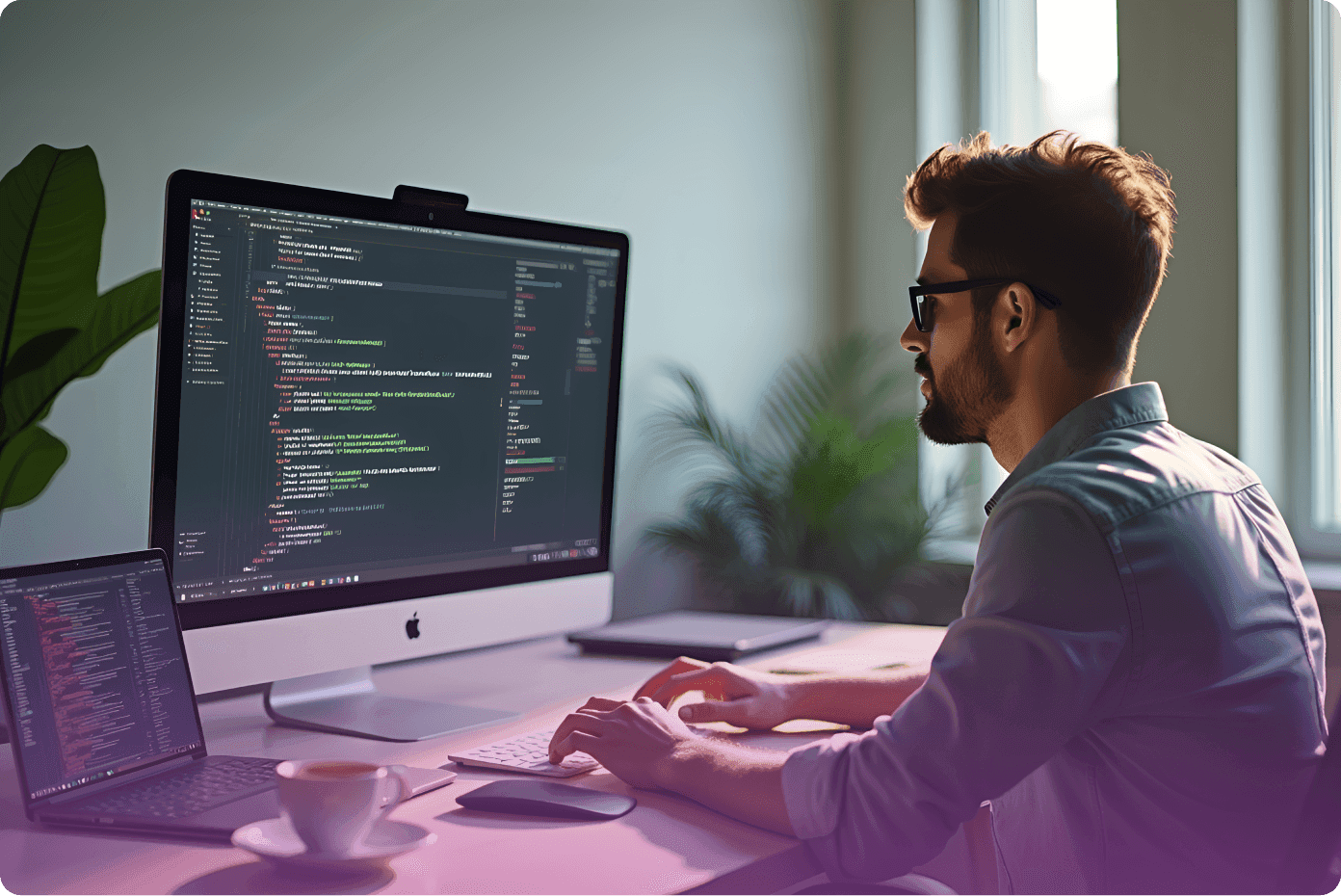
App Development
FuturByte offer a full cycle of app development services which includes design, integration and maintenance, from ideation to final deployment. Our aim is to understand your requirements and provide you with an application designed specifically for your business.

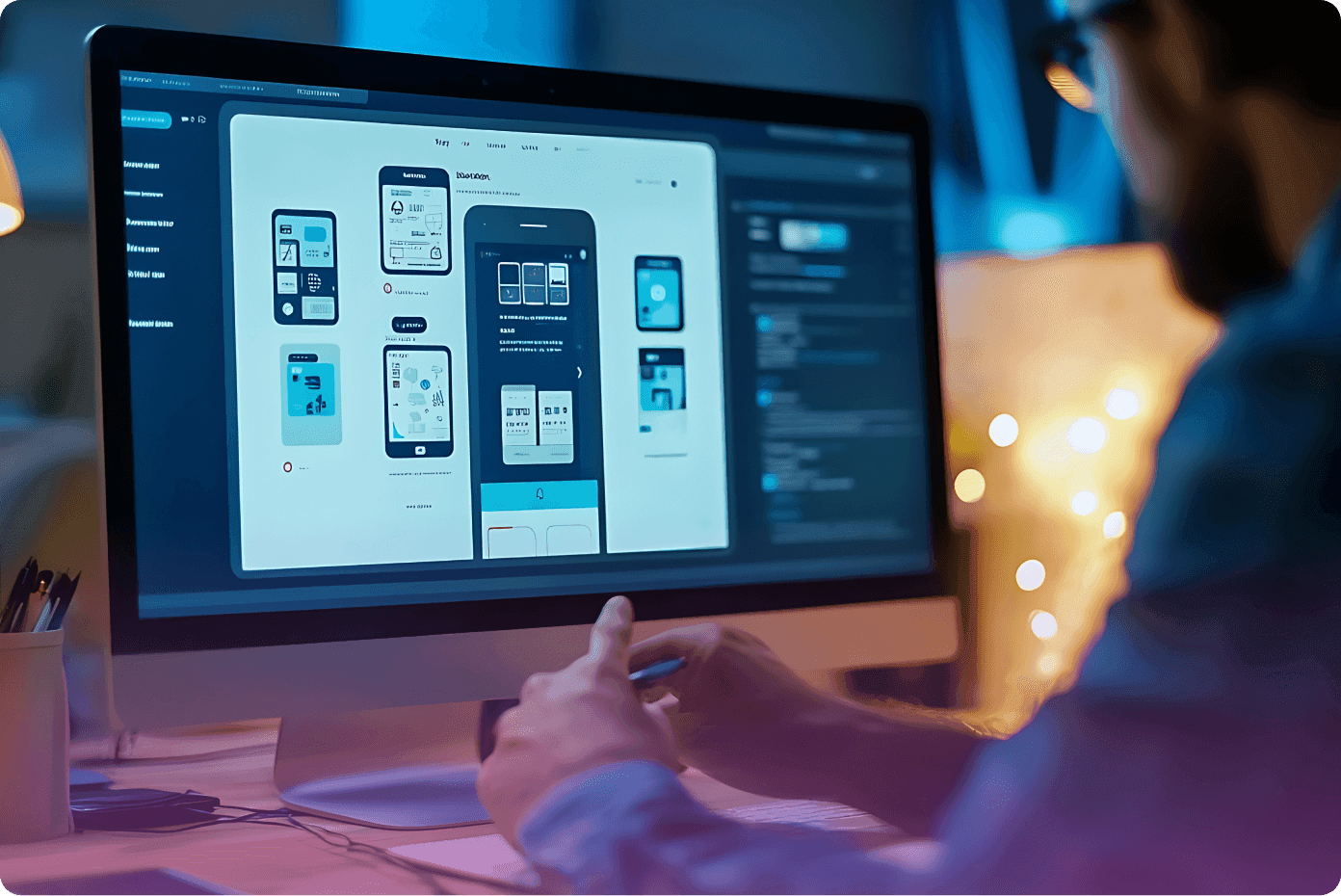
Business Management Solutions
We create business management solutions keeping the needs of our customers in mind. We will work with you to understand how you will be using the solution and work accordingly to provide you with a premium solution.

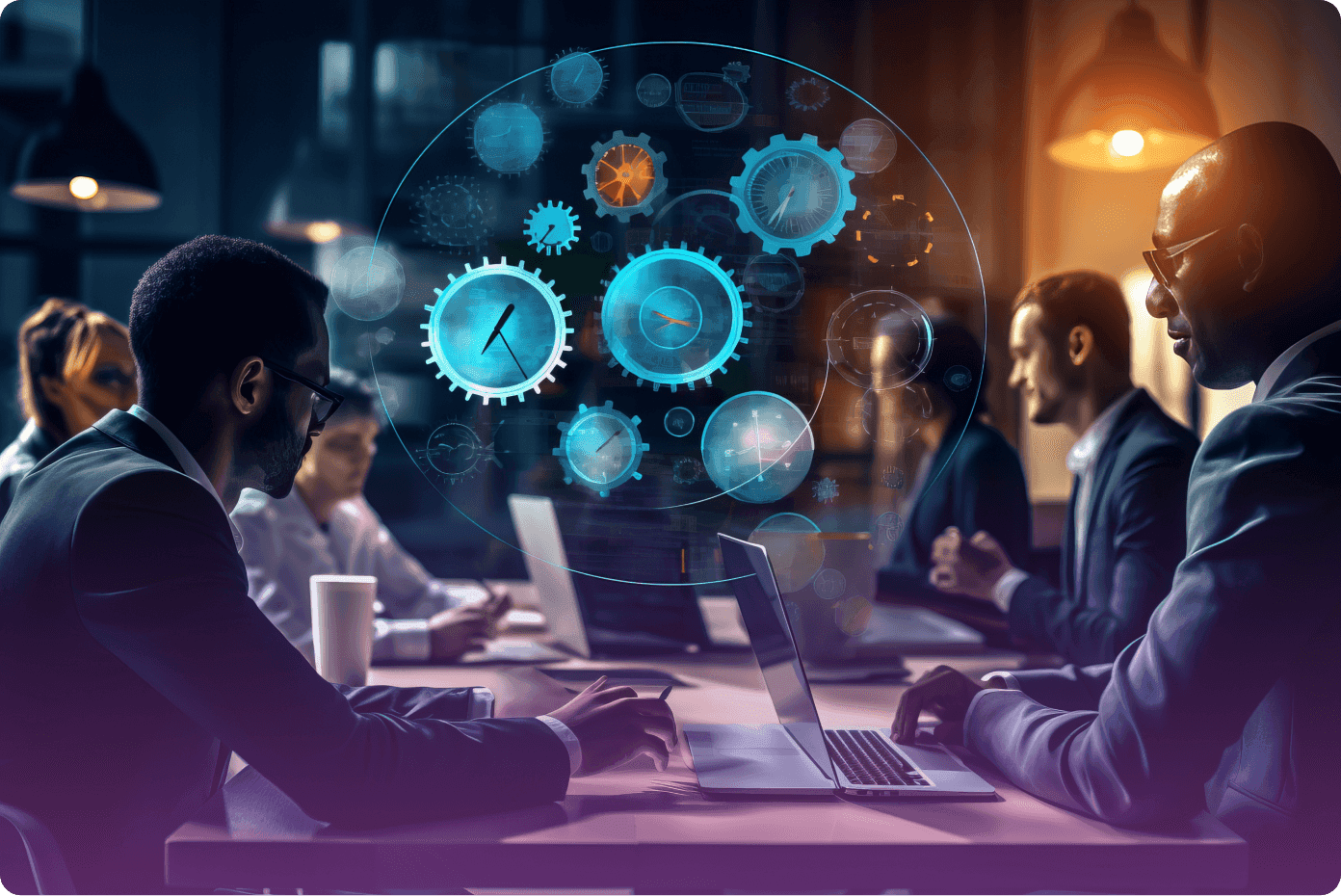
E-Commerce
Our experts at FuturByte have been helping online businesses expand their reach and gain new customers for years. Contact us today and kickstart your e-commerce journey with us.

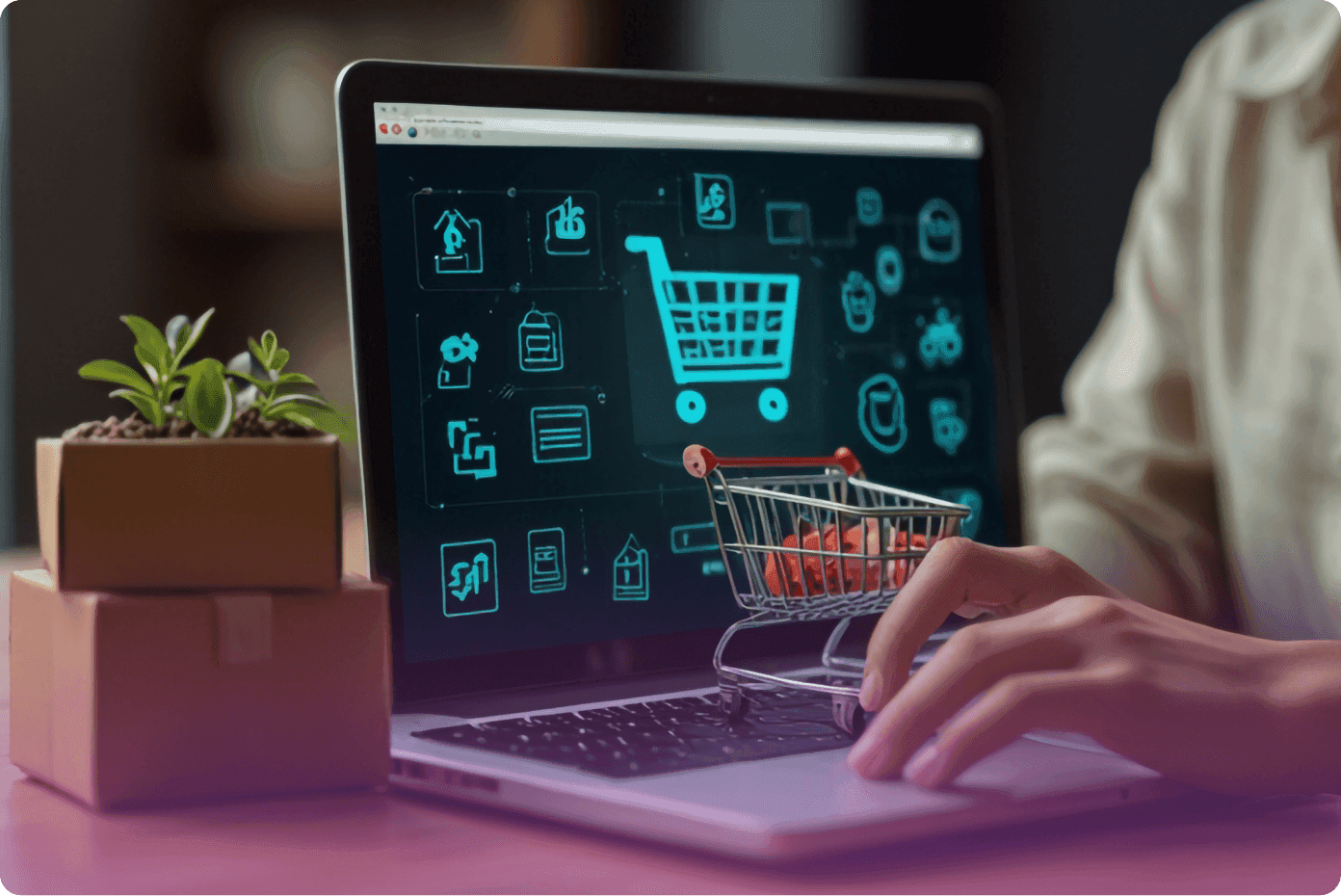
Cloud Solutions
FuturByte places a premium on both efficiency and innovation. We recognise that speed alone is not enough. We strategically integrate Cloud Development services to not only accelerate development cycles but also ensure sustained, long-term growth for businesses.

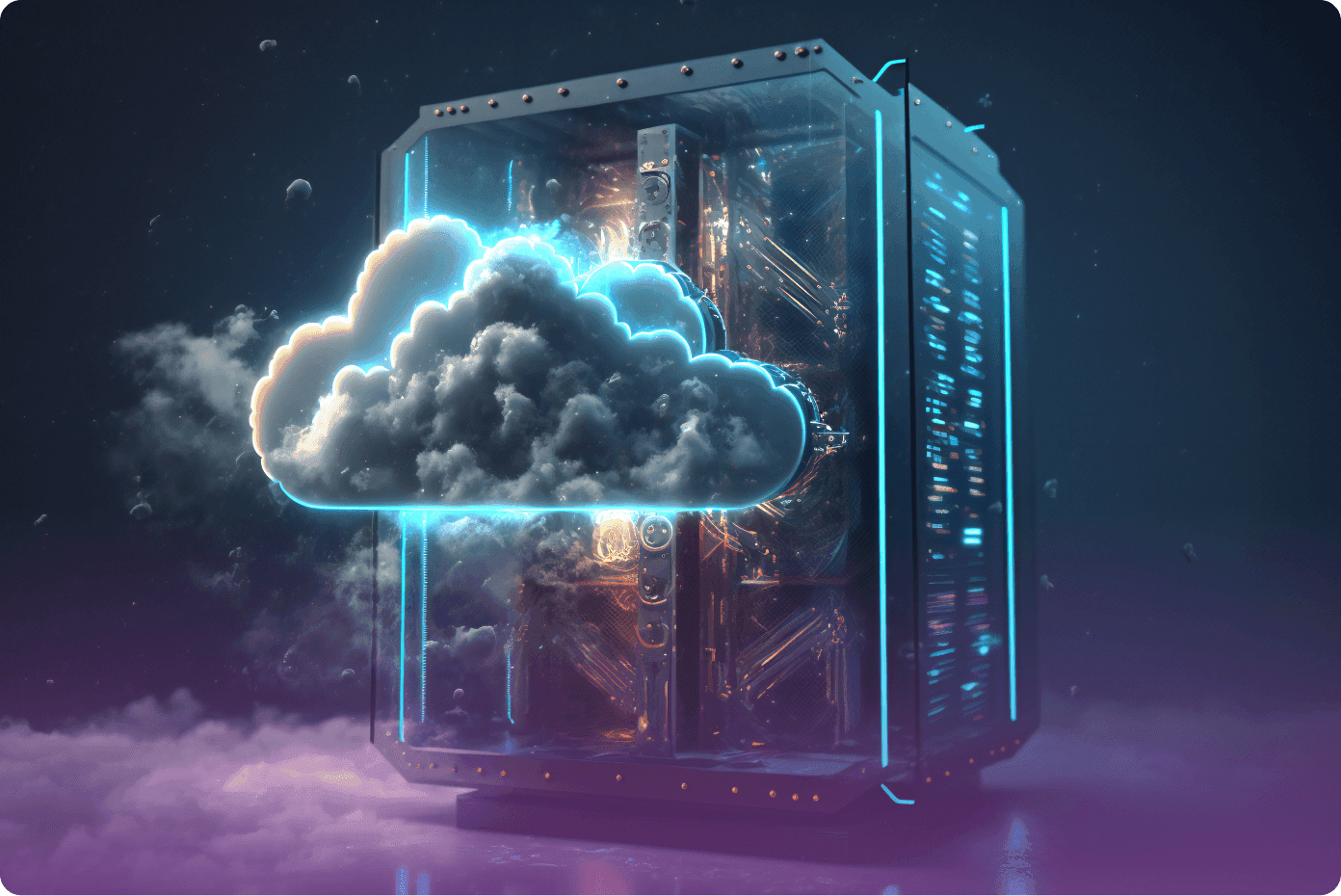
SEO Services
FuturByte plan data-driven SEO strategies that put your website at the forefront of global search results, attracting customers across borders and skyrocketing your sales. Don’t settle for local limitations. Partner with FuturByte and conquer the global market.

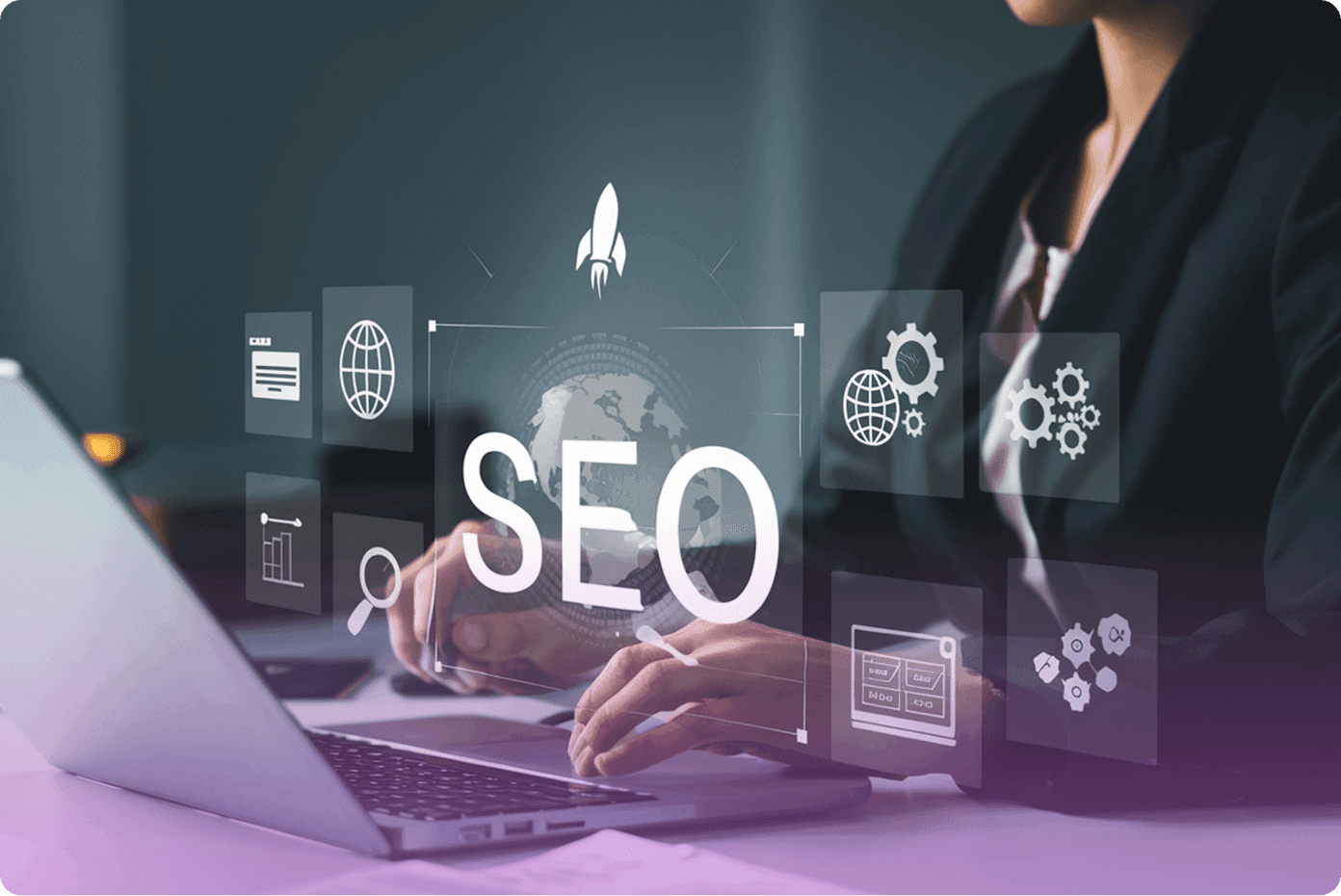
Custom Digital Solutions Built from Scratch
Every B2B business is unique, so one-size-fits-all software won’t do. Our custom software solutions are built from scratch to solve your problems effectively.
Smart Solutions, No Templates
We create fully customised digital solutions, designed to match the exact requirements of our clients, streamlining their operations and improving productivity. We don't believe in template-based or readymade solutions. Our approach is to first understand the challenges and goals of our clients and then design solutions that perfectly fit.
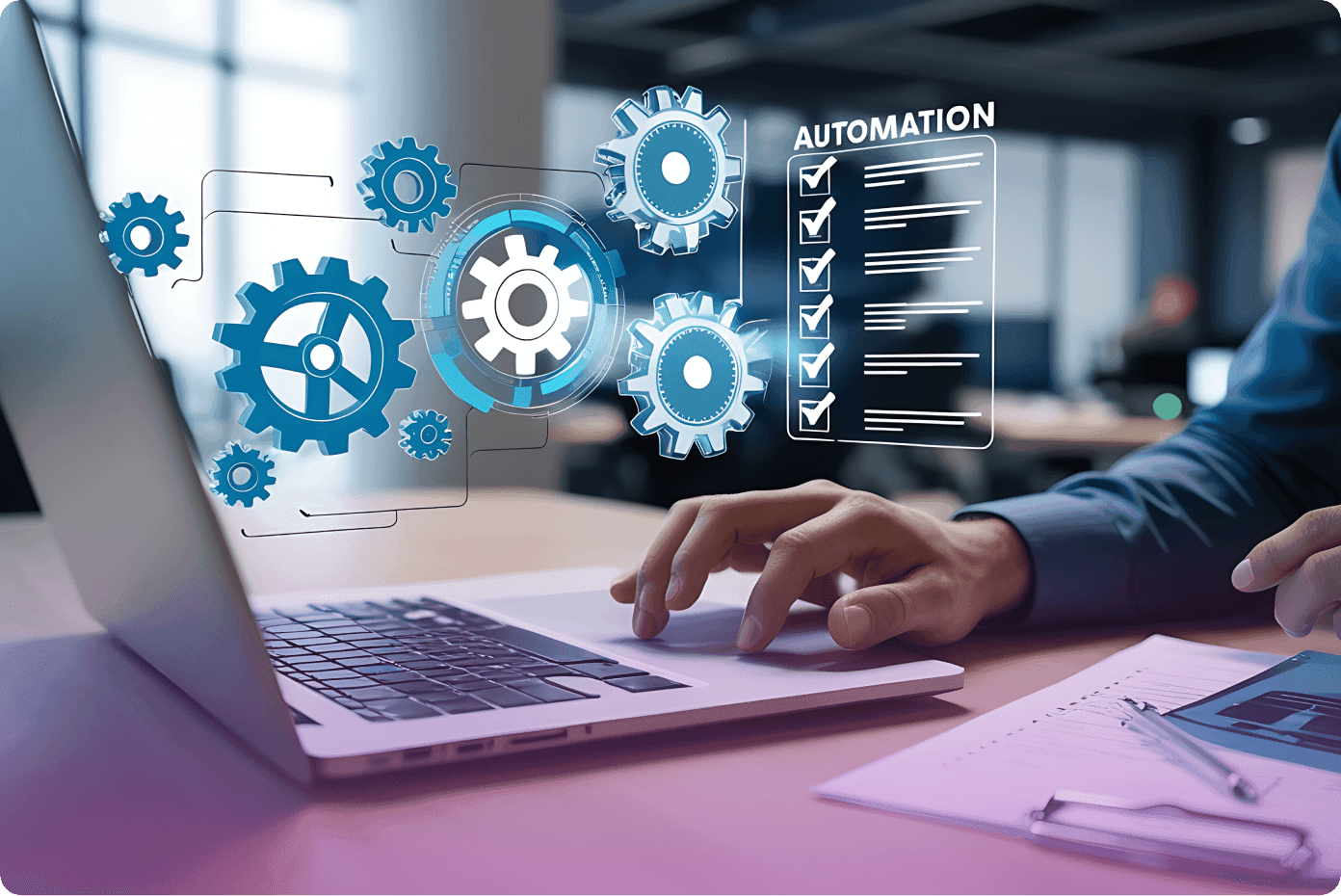
Future-Ready System Upgrades
We modernise outdated systems, integrating the latest technologies to ensure seamless performance. Our solutions help enhance efficiency, reliability, and scalability, providing businesses with the tools needed to thrive in today’s fast-paced business landscape.
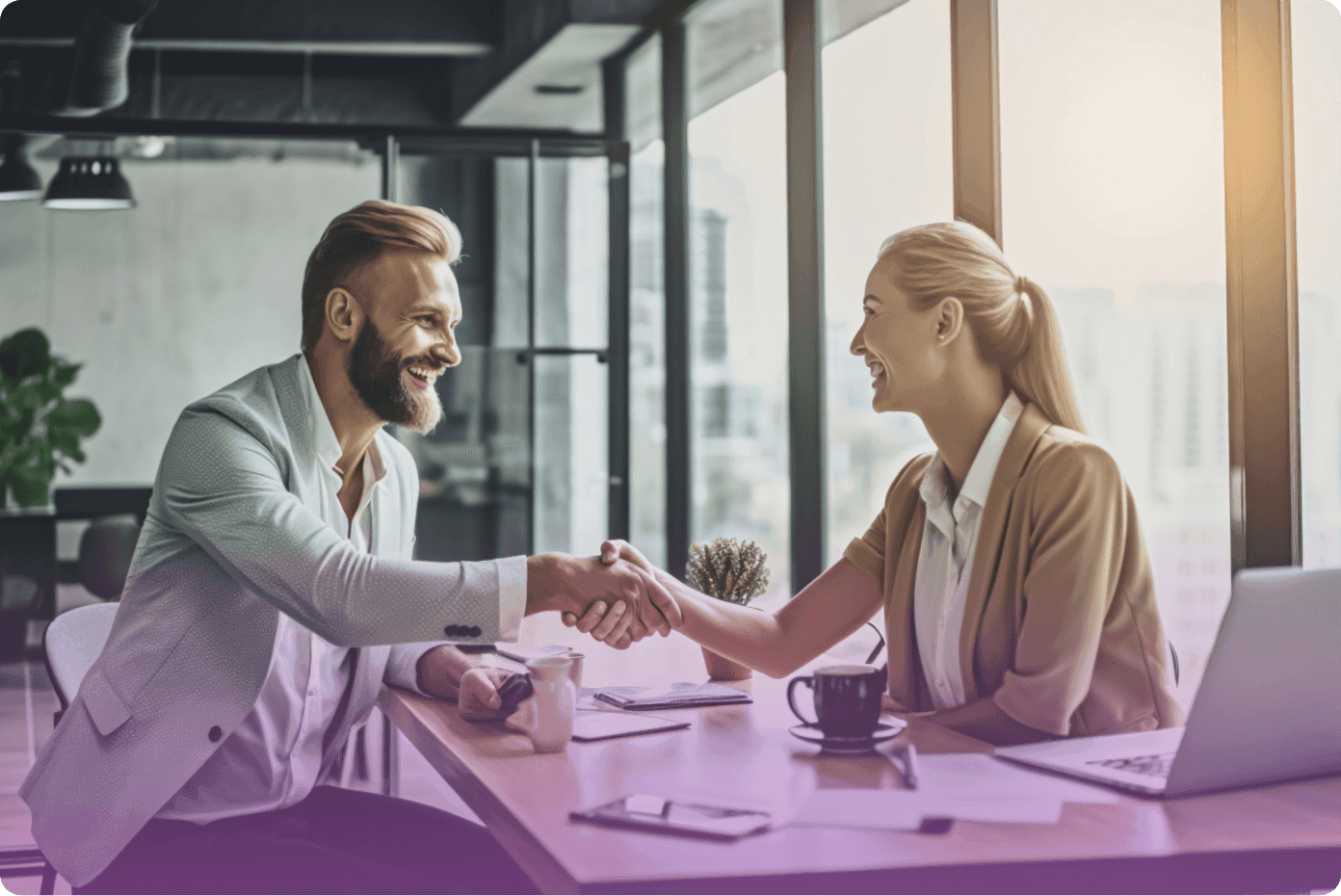
Developing 100% Safe & Secure Solutions
We build software solutions with top-notch security, ensuring the data is safeguarded with advanced encryption, secure coding practices, and rigorous testing. We prioritise data security, reliability, and peace of mind for your business - and extending this sense of security to your clients.
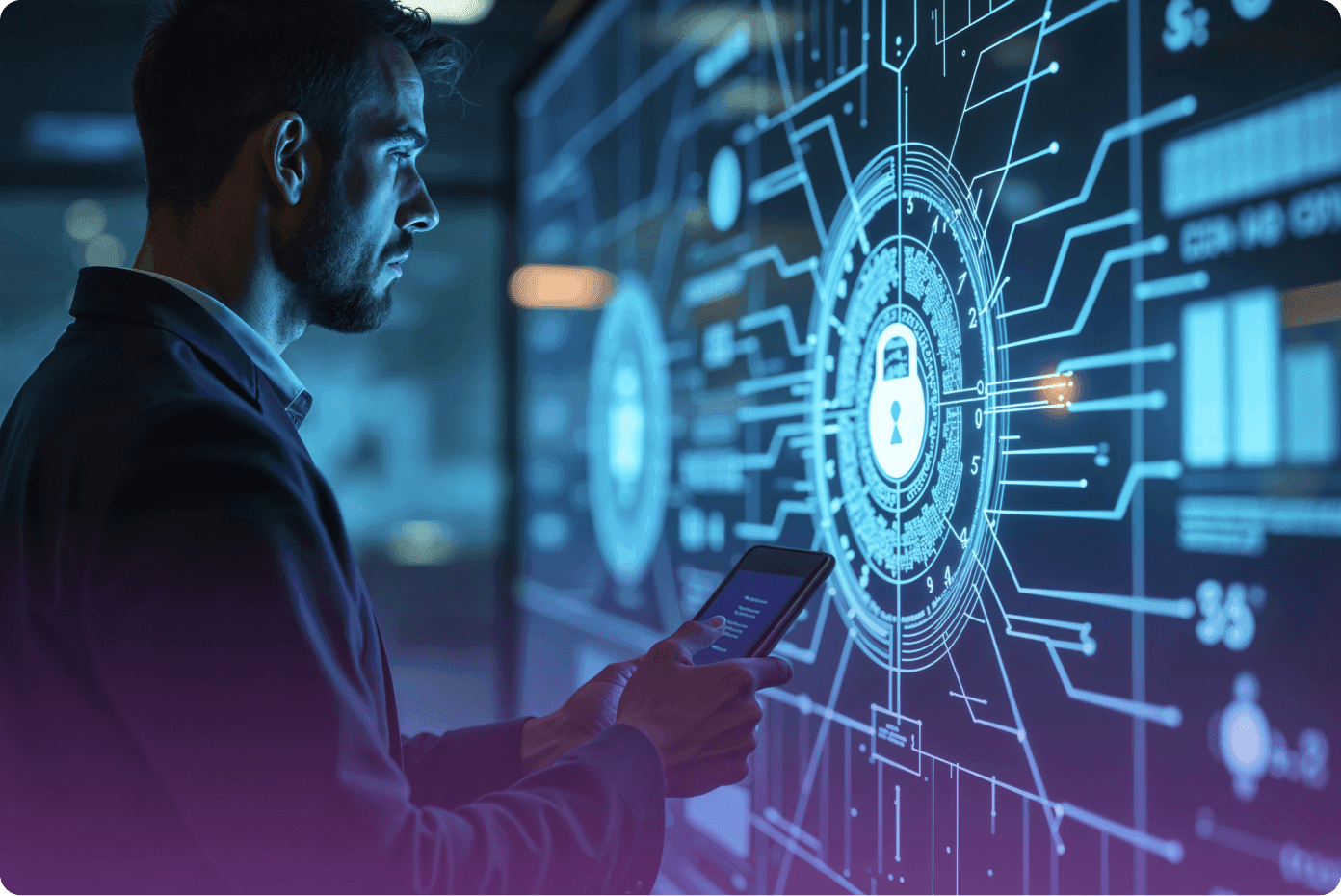
Our Expertise
IOS




Android





Technologies

CMS Platforms

Mobile Frameworks


Back-End Frameworks

Popular Ecommerce Platforms




Open-Source Solutions

Relational Databases



NoSQL Databases

Cloud Providers


Containerisation


CI/CD Tools


Let’s schedule a call to discuss your project.
Every B2B business is unique, so one-size-fits-all software won’t do. Our custom software solutions are built from scratch to solve problems effectively.
Schedule Free Consultancy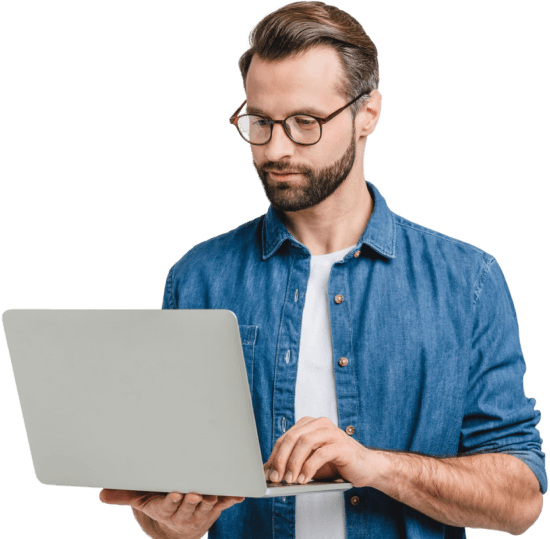
FuturByte’s Edge in Digital Innovation
B2B Specialisation
We understand the complexities of B2B industries and have developed a proven process to ensure our solutions meet the unique challenges faced by businesses like yours.
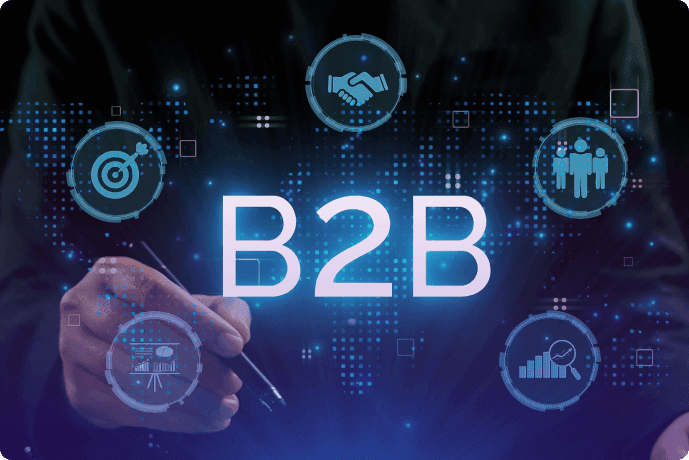
Collaborative Approach
We will work closely with your team to understand your requirements and business, allowing out to develop solutions that continue to deliver value long after the launch too.
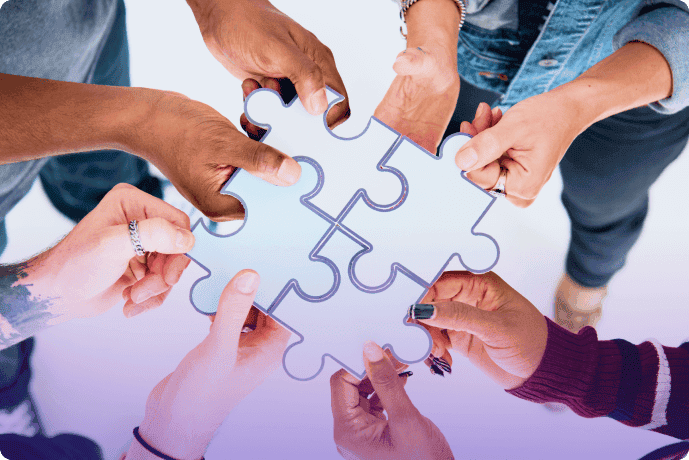
Expertise in Digital Solutions
We don’t just deliver software. We deliver digital solutions that are strategically aligned with the goals of our clients. Our team has extensive experience in developing custom solutions that solve real-world problems and we are ready to now build yours.
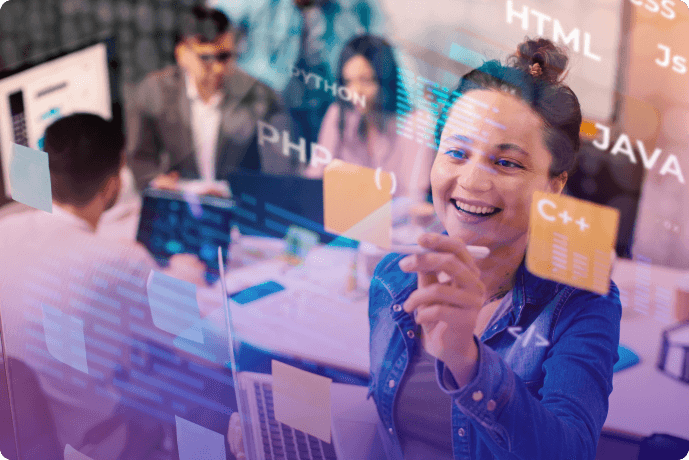
Focused on Results
We are committed to delivering measurable results. Whether it's increasing operational efficiency, enhancing customer satisfaction, or boosting sales, our solutions are designed to drive success.
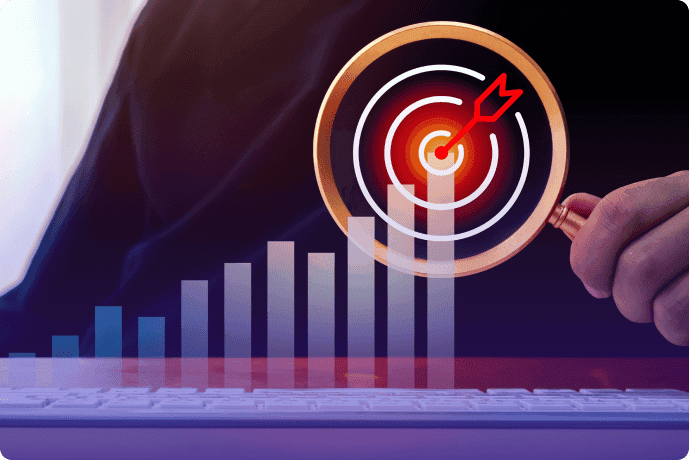
What our customers are saying
Let’s Connect!
Get in touch with us to discuss your project ideas and goals. Our team will take it from there.
Open Communication
Customer-Centric
Transparency
Industry Experts
Continuous Innovation
Reliable

Let's connect to discuss your project.
Fill out the below form